Comparing Asynchronous Programming in Python and Rust
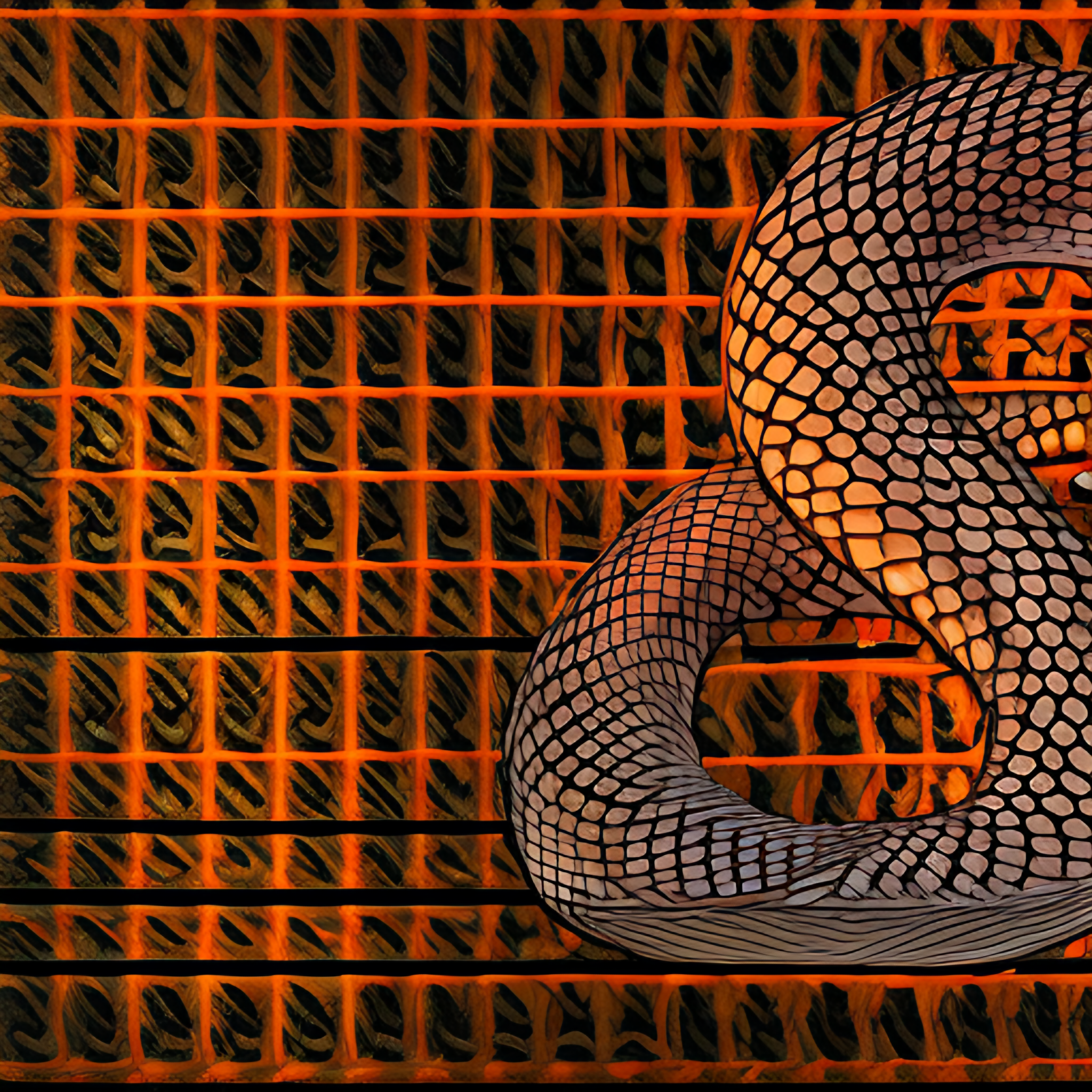
Asynchronous programming is a programming paradigm that allows you to write code that can perform multiple tasks concurrently, without using threads. Both Python and Rust have support for async programming, but the approaches to async programming in these languages are quite different.
Async Programming in Python
In Python, asynchronous programming is implemented using the asyncio
module, which is part of the Python standard library. The asyncio
module provides a number of tools for writing asynchronous programs, including the async
and await
keywords, which allow you to define asynchronous functions and await the results of asynchronous operations.
Here’s an example of an asynchronous function in Python:
import asyncio
async def foo():
print("Starting foo")
await asyncio.sleep(1)
print("Finished foo")
In this example, the foo function is an asynchronous function that prints a message, waits for one second using the asyncio.sleep function, and then prints another message. To run this asynchronous function, you would use the asyncio.run function, like this:
asyncio.run(foo())
This would execute the foo function asynchronously, and you would see the messages “Starting foo” and “Finished foo” printed to the console.
Async Programming in Rust
Rust also has support for async programming, through the use of the async and await keywords and the futures crate. The futures crate is a library for writing async programs in Rust, and it provides a number of tools for defining async functions, awaiting async operations, and managing async tasks.
Here’s an example of an async function in Rust:
use async_std::task;
use futures::future::{self, Future};
async fn foo() -> i32 {
println!("Starting foo");
task::sleep(std::time::Duration::from_secs(1)).await;
println!("Finished foo");
42
}
In this example, the foo function is an async function that prints a message, waits for one second using the task::sleep function, and then prints another message. To run this async function, you would use the future::block_on function, like this:
let result = future::block_on(foo());
This would execute the foo
function asynchronously, and the value 42 would be stored in the result variable.
Comparison
Overall, both Python and Rust have support for async programming, but the approaches to async programming in these languages are quite different. Python’s asyncio module provides a more high-level interface for writing async programs, while Rust’s futures crate provides a more low-level interface that gives you more control over the details of the async execution.
Which language is better for async programming depends on your specific needs and preferences. Python’s asyncio
module may be easier to use for simple async programs, while Rust’s futures crate may be more powerful and flexible for more complex async programs.