Maximizing Performance with Compiled Extensions in Python
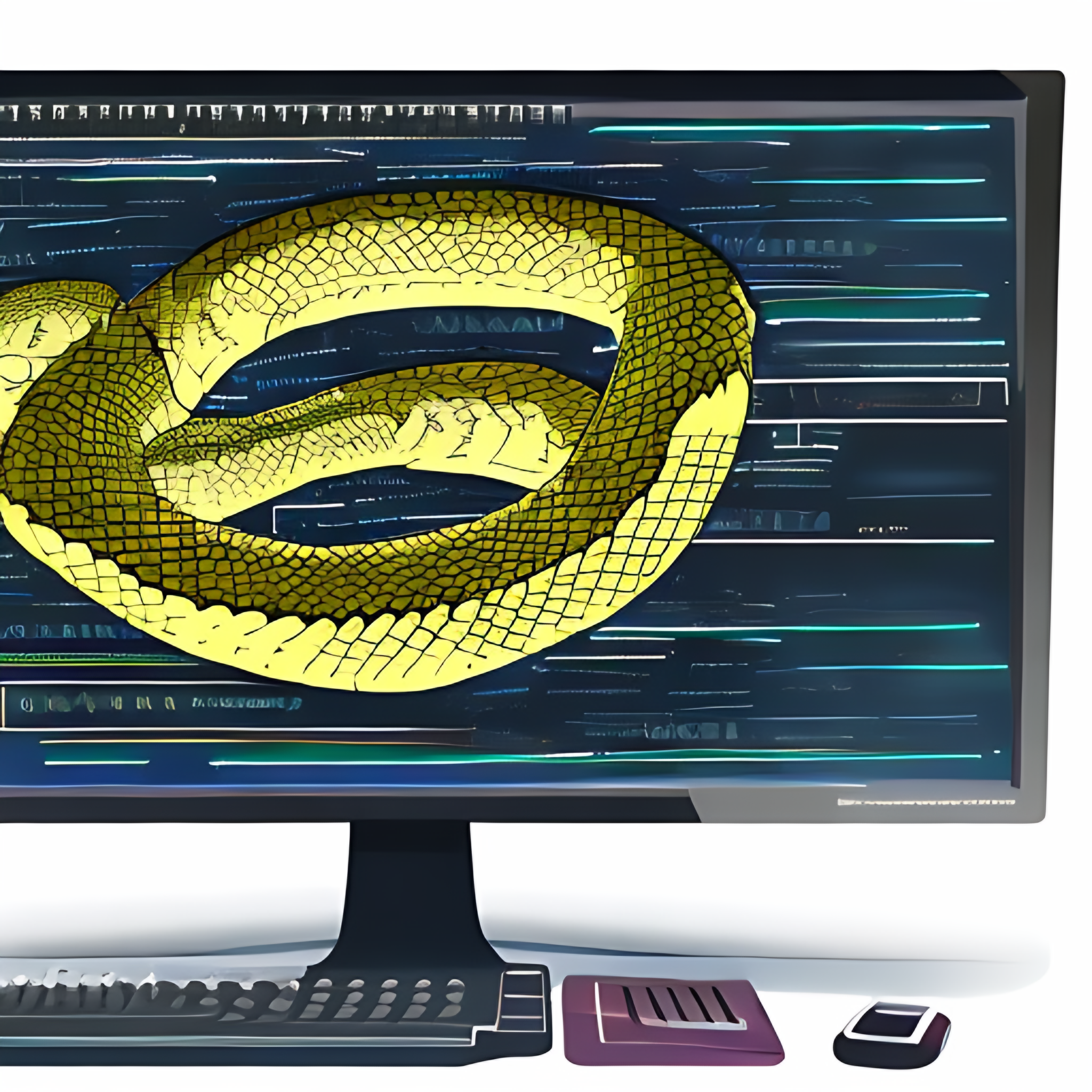
Compiled extensions are a way to extend the functionality of Python by adding code written in a compiled language such as C or C++. These extensions can be used to improve the performance of certain tasks, interface with existing C libraries, or provide access to functionality that is not available in pure Python. In this article, we will explore the process of creating and using compiled extensions in Python, including the tools and techniques you will need to get started.
Why Use Compiled Extensions?
There are several reasons why you might want to use compiled extensions in Python:
Performance: One of the main motivations for using compiled extensions is to improve the performance of certain tasks. Python is an interpreted language, which means that it is relatively slow compared to compiled languages like C or C++. By writing critical code in a compiled language, you can often achieve much better performance.
Access to C libraries: Another reason to use compiled extensions is to interface with existing C libraries. This can be particularly useful if you need to use a library that has not been wrapped in a Python-friendly form, or if you want to use a library that is only available in C.
Functionality not available in Python: Finally, compiled extensions can be used to provide access to functionality that is not available in pure Python. This might include hardware-specific functionality, low-level system calls, or other types of functionality that are difficult or impossible to implement in Python.
Setting Up Your Development Environment
Before you can start working with compiled extensions in Python, you will need to set up your development environment. This includes installing a C or C++ compiler, as well as any other tools and libraries that you will need.
Installing a Compiler
The first step in setting up your development environment is to install a C or C++ compiler. There are many different compilers available, including GCC (GNU Compiler Collection) and Clang. On Windows, you can use Microsoft Visual C++.
To install GCC on a Unix-based system (such as Linux or macOS), you can use your system’s package manager. For example, on a Debian-based system, you can use the following command:
sudo apt-get install build-essential
On macOS, you can install GCC by installing the Xcode command line tools:
xcode-select --install
On Windows, you can download and install Microsoft Visual C++ from the Microsoft website.
Installing Other Tools and Libraries
In addition to a compiler, you may also need to install other tools and libraries depending on your specific needs. For example, you may need to install libraries for working with specific hardware or for interfacing with particular C libraries. You may also need to install tools for debugging and testing your compiled extensions.
Writing a Compiled Extension
Once you have your development environment set up, you can start writing a compiled extension for Python. The basic steps for creating a compiled extension are:
-
Write the extension module in C or C++.
-
Compile the extension module into a shared library using a C or C++ compiler.
-
Use the import statement in Python to load the compiled extension module.
Let’s take a closer look at each of these steps.
Writing the Extension Module
The first step in creating a compiled extension is to write the extension module in C or C++. An extension module is simply a C or C++ file that contains the functions and other code that you want to make available to Python.
Here is a simple example of a Python compiled extension written in C:
#include <Python.h>
// A function that adds two integers and returns the result
static PyObject* add(PyObject* self, PyObject* args) {
// Parse the arguments
int a, b;
if (!PyArg_ParseTuple(args, "ii", &a, &b)) {
return NULL;
}
// Return the result
return PyLong_FromLong(a + b);
}
// A function that multiplies two integers and returns the result
static PyObject* mul(PyObject* self, PyObject* args) {
// Parse the arguments
int a, b;
if (!PyArg_ParseTuple(args, "ii", &a, &b)) {
return NULL;
}
// Return the result
return PyLong_FromLong(a * b);
}
// A function that calculates the factorial of a number
static PyObject* fact(PyObject* self, PyObject* args) {
// Parse the arguments
int n;
if (!PyArg_ParseTuple(args, "i", &n)) {
return NULL;
}
// Calculate the factorial
int result = 1;
for (int i = 2; i <= n; i++) {
result *= i;
}
// Return the result
return PyLong_FromLong(result);
}
// A table of function definitions
static PyMethodDef methods[] = {
{"add", add, METH_VARARGS, "Add two integers"},
{"mul", mul, METH_VARARGS, "Multiply two integers"},
{"fact", fact, METH_VARARGS, "Calculate the factorial of a number"},
{NULL, NULL, 0, NULL}
};
// The module definition
static struct PyModuleDef module = {
PyModuleDef_HEAD_INIT,
"example", // Module name
NULL, // Module documentation
-1, // Module state (negative means it is global)
methods // Module methods
};
// The initialization function
PyMODINIT_FUNC PyInit_example(void) {
return PyModule_Create(&module);
}
To compile this code into a Python module, you would need to use a C compiler such as GCC. For example, you could use the following command:
gcc -shared -o example.so -fPIC example.c `python3-config --cflags --ldflags`
Compiling on arm64 macOS
To compile a C extension for Python on arm64 macOS, you will need to install a C compiler and use it to build the extension.
Once the Xcode command line tools are installed, you can use the Clang compiler to build your C extension. To do this, you will need to create a C source file that contains your extension code and a setup.py script that tells Python how to build and install the extension.
Here is an example of a simple setup.py script that can be used to build a C extension:
from distutils.core import setup, Extension
# Define the extension module
module = Extension('example', sources=['example.c'])
# Run the setup function
setup(name='example',
version='1.0',
description='An example C extension for Python',
ext_modules=[module])
To build the extension, open a terminal and navigate to the directory containing the setup.py script and your C source file. Then, run the following command:
python3 setup.py build
This will build the extension and create a shared library file in the build directory. To install the extension, run the following command:
python3 setup.py install
This will install the extension and make it available for use in Python.
Then, you can use the import statement in Python to import the compiled extension module:
import example
# Call the add function
result = example.add(1, 2)
print(result) # 3
# Call the mul function
result = example.mul(2, 3)
print(result) # 6
# Call the fact function
result = example.fact(5)
print(result) # 120
Debugging Compiled Extensions
Debugging compiled extensions can be a challenge, especially if you are not familiar with C or C++. Some tools and techniques that can be helpful for debugging compiled extensions include:
- Using a debugger such as GDB or LLDB to step through your code and examine variables
- Printing debugging output to the console using printf or cout
- Wrapping your C or C++ functions in Python functions and calling them from Python, which can make it easier to debug using Python’s built-in debugging tools
Testing Compiled Extensions
It is important to test your compiled extensions to ensure that they are working correctly. You can use the same techniques for testing compiled extensions as you would for any other code, including unit testing and integration testing.
Distributing Compiled Extensions
When you are ready to distribute your compiled extension, you will need to provide a way for users to install it. One option is to package your extension as a Python module using tools like setuptools or distutils. This will allow users to install your extension using the pip package manager.
Alternatively, you can distribute your compiled extension as a standalone library that users can link against. In this case, you will need to provide instructions on how to build and install the library.
In either case, it is important to provide clear documentation on how to use your compiled extension, including any dependencies and any necessary configuration steps.
Conclusion
Compiled extensions are a powerful way to extend the functionality of Python and improve the performance of certain tasks. By following the steps outlined in this article, you can create and use compiled extensions in Python to access functionality not available in pure Python, interface with existing C libraries, or improve the performance of your code.