Five Strategies for Speeding Up Your Python Code
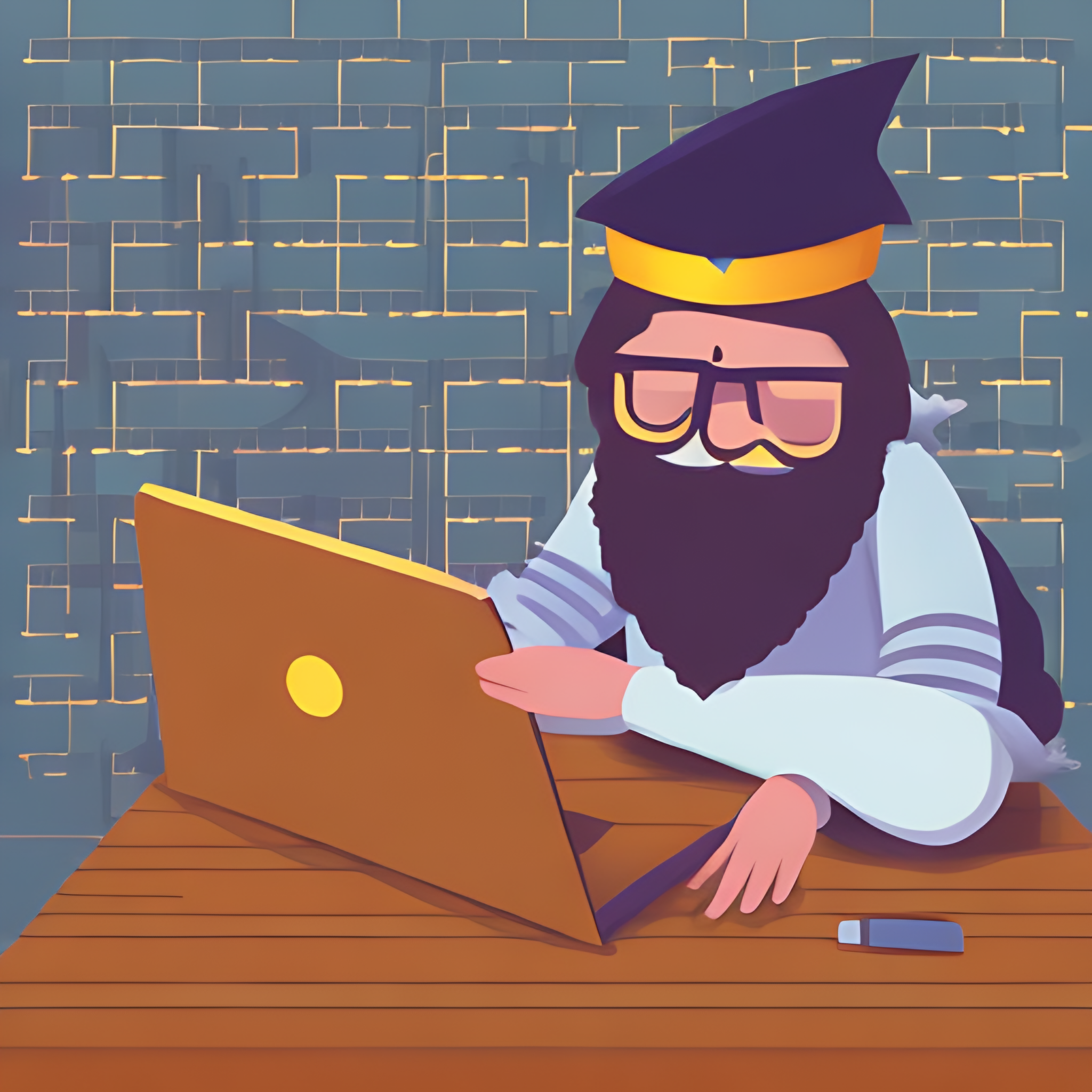
Python is a powerful and versatile programming language, but it is not always the fastest option for performance-critical tasks. If you find that your Python code is running slower than you would like, there are a number of strategies you can use to speed it up. Here are five effective techniques to consider:
Use compiled extensions
If you have a performance-critical section of code, you can try writing it in a compiled language (such as C or Cython) and using it as an extension in Python. This can provide a significant speed boost for CPU-intensive tasks. To use compiled extensions in Python, you will need to install a C compiler and use a tool like distutils or setuptools to build and install the extension.
Use parallelism
If you have a CPU-intensive task that can be parallelized, you can use the multiprocessing or concurrent.futures modules to run the task concurrently on multiple CPU cores. This can be an effective way to speed up your code by taking advantage of multiple CPU cores.
Use vectorization
If you have a task that involves performing the same operation on many items in a list or array, you can try using vectorized operations from the numpy library. Vectorized operations can be much faster than looping over the items in the list and performing the operation on each item individually.
Use optimized data structures
Python provides a number of optimized data structures, such as array.array and collections.deque, which can be faster than using a list in certain situations. For example, the array.array data type is a more memory-efficient alternative to a list for storing large arrays of numerical data.
Use profiling tools
Profiling tools, such as the cProfile module or the profile decorator, can help you identify the parts of your code that are taking the most time to execute. This can help you focus your optimization efforts on the most important areas.